Redis is an incredible key-value database that is fast because it stores data in the system's memory instead of the much slower disk.
It is a popular choice for high-performance applications and can be used as a standalone database or a caching system for other databases.
Despite being a key-value store, it supports more data types other than strings as its values. For keys, Redis supports only binary-safe string values with a maximum size of 512 MB.
A binary string refers to a sequence of bytes used in storing non-traditional data such as images. Unlike raw strings, which contain text information, binary strings can store data of various formats.
In this detailed article, we will discuss the data types supported in Redis. We then look at how to use the Redis commands to work with the discussed data types.
Let us discuss.
Redis Data Types
Redis is a key-value store, works by mapping a unique key to a specific value object. Using each individual key in the database, you can retrieve, update, or remove the value associated with the key.
This feature makes Redis very easy to use and manage. As mentioned, keys in the Redis database are binary strings. However, for values, you can use various objects such as:
- Strings
- Lists
- Hashes
- Sets
- Sorted Sets
- BitMaps (not discussed)
- HyperLogLog (not discussed)
Redis provides a set of commands and operations for each data type mentioned above. To follow along with this tutorial, ensure you have a Redis cluster setup on your system and ready to go.
String Types
String types are the most basic and standard unit types you can set to a Redis key. As mentioned, a Redis key has a maximum size of 512 MB either in text or binary string. However, it is recommended to set shorted keys.
Working with strings in Redis is very straightforward and tremendously fast. To work with Redis strings, use commands such as GET, SET, and DEL.
To add a new key to the Redis database, open the Redis CLI and enter the command following the syntax shown below:
SET key value
The set command takes the first and second arguments as the key and value, respectively.
To fetch the value stored in a specific key, use the GET command followed by the name of the key.
For example:
GET key
"value"
Once you run the command above, you should see the value stored by a specific key.
To delete a key and value from the database, use the DEL command followed by the name of the key.
DEL key
Output: (integer) 1
Once you execute the command, Redis will return the number of elements removed from the database.
If none of the keys specified exists on the database, Redis will return 0, as shown in the example below:
DEL notthere
Output:
(integer) 0
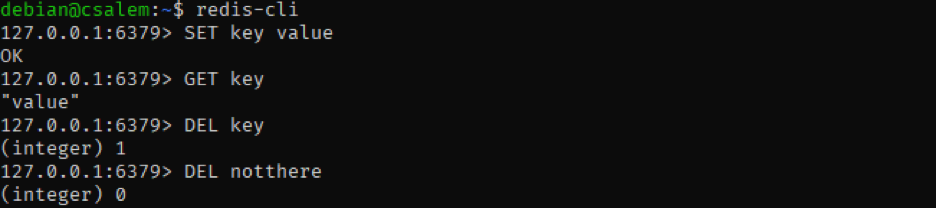
List Types
Redis also supports lists. Lists represent an ordered sequence of string values associated with a specific key. Think of Lists as an ordered collection of string values.
There are various upsides and downsides to using Lists in Redis. First, lists provide a quick method to insert and remove elements from the head.
The drawback to the list is that when we need to access an element from the collection, Redis will have to scan the entire group. This becomes a disadvantage, especially if read operations are higher compared to write operations.
In Redis, you can add elements to a list by pushing it to the left – meaning adding it to the head of the list or pushing it to the right – to the tail.
The following are the commands when you need to work with Lists in Redis.
To create a new List, use either the LPUSH
or RPUS
H command.
The LPUSH
will add a new element to the head of the specified list, while the RPUSH
will add the element to the tail of the list.
LPUSH databases MongoDB
RPUSH databases MySQL
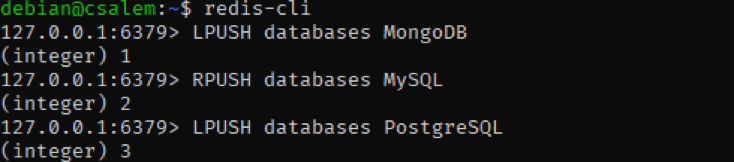
To retrieve a specific range of items, use the LRANGE
command followed by the start and stop values.
For example, to get the first 4 values, we can use the LRANGE
command as shown below:
LRANGE databases 0 3

The command should return the elements from index 0 to index 4.
If you want to remove elements from a Redis list, you can use the LPOP
and RPOP
commands. The LPOP
will remove the elements from the head, while RPOP
will delete elements from the tail.
LPOP databases
"Redis"
The LPOP/RPOP
commands will return the value of the element removed from the list.
RPOP databases
"Firebase"

Suppose you want to get a value from a specific index position in the list. You can us the LINDEX command as:
LINDEX databases 2
"MySQL
It is good to note that there are more commands to use with Lists than those discussed in this tutorial. Consider the documentation as provided in the link below.
Hash Types
Redis also supports Hashes as a data type. We can define a hash as a collection of key-value pairs. In most cases, hashes can be helpful in mapping string fields and values.
For example, let us say we need to store the patient information and the level of the illness. We can set the condition as the key and the values as a set of key-value pairs with the patient's name and the level of illness.
The following are basic commands you can use to work with Hashes in Redis.
To create a Hash, use the HSET
command. This command maps the key within a specified hash.
HSET illness_1 "John Doe" 2
In the example above, we create a hash where the illness_1 is the key.
To get a value associated with a key in the hash, use the HGET
command followed by the name of the hash and the specific key.
For example:
HGET illness_1 "Bruce K"
"1"
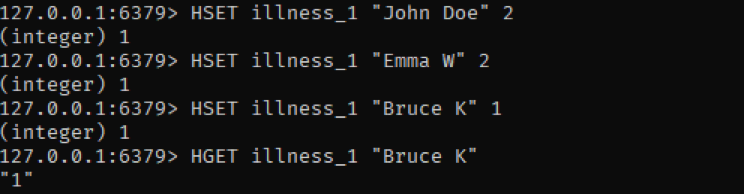
To view the complete hash, use the HGETALL command as shown:
HGETALL illness_1

The command should return the specific keys and values within the hash as shown in the screenshot above.
To remove a specific key-value pair from the hash, use the HDEL
command followed by the hash name and the key to remove.
For example, let us remove the information about John Doe from the hash. We can do:
HDEL illness_1 "John Doe"
(integer) 1
The command will return the total number of items removed. In our example, only one key-value pair is removed.
Set Types
The following data type supported by Redis is the set. A set is very similar to a list with one significant difference: a list does not allow duplicate values.
Hence, we can define a set as a collection of unique unordered string values. Sets are not ordered. This removes the ability to add or remove items left or right of the index. Sets, however, are instrumental when you need to store unique values.
The following are commands provided by Redis to work with Sets.
To create a new set, use the SADD command followed by a key and the value to store.
SADD my-key member1
(integer) 1
To get all the members within a set, use the SMEMBERS
command:
SMEMBERS my-key
1) "member4"
2) "member2"
3) "member3"
4) "member1"
5) "member5"
To find out if an item is a member of a set, use the SISMEMBER
command:
SISMEMBER my-key member3
(integer) 1
The command returns one if a specified member exists within a set.
However, adding a member to a set multiple times will always produce the same result.
To remove a member from a set, use the SREM
followed by the key and the member to remove.
SREM my-key member1
(integer) 1
The command above should return an integer value indicating the number of elements deleted.
Sorted Set Types
Sorted sets are one of the functional and advanced data types in Redis. A sorted set is comprised of three main components. The first is the unique string which acts as the key. The second is a member and a third value which is known as a score.
Each element in a sorted set is mapped to a floating-point value (score) which is then used to sort them in various orders.
The following are basic commands to interact with sorted sets in Redis.
To add a member to a sorted set with a score, use the ZADD
command.
ZADD mykey 1 member1
The items after the ZADD
command represent the key, score, and member, respectively.
To fetch the items based on their position in the set, use the ZRANGE
command:
ZRANGE mykey 0 100

To remove an item from the sorted set, use the ZREM
command:
ZREM mykey member6
Similarly, the command should return an integer value indicating the number of items removed from the sorted set.
Final Thoughts
And with that, we have concluded our tutorial. In this guide, you learned how to work with various data types in Redis using a set of commands.