Python uses indentation, unlike other programming languages that use curly braces to define a code block. Therefore, ensuring a strict and correct indentation format in your Python program is essential.
Note: We refer to the space insert at the beginning of a code line when we refer to indentation. Therefore, indentation is more than a part of readability concerns in Python.
What is an indentation, and How do we use it in Python?
Indentation refers to the space added at the beginning of a line. We most accomplish this by adding a whitespace character before a specific code block.
According to Python Formatting rules, an indentation block should be done using four space characters per indentation level.
Cause of IndentationError: Unindent does not match any outer indentation level.
Python recommends that you use four space characters per code level.
However, if you are working on a code project that uses tabs, you should continue using tabs throughout the program.
Mixing both tabs and space characters as indentation characters will lead to Python throwing the IndentationError. Unindent does not match any outer indentation level error
.
Fix: Mixing Tabs and Space Characters.
Consider the example code shown below:
for i in range(5):`
print(i) # spaces
print(i) # tabs
In the example above, we have two indentations, one that uses space characters and the other that uses tabs.
If we run the code above, we should get an error as shown:
IndentationError: unexpected indent
To fix this issue, use your editor formatting features to search for all tabs characters and replace them with spaces.
In Vim, you can enter command mode and run:
gg=G
In Visual Studio code, launch the command palette by pressing the keys CTRL + SHIFT + P
.
Next, search for Convert indentation to (and select tabs or spaces)
depending on your project format.
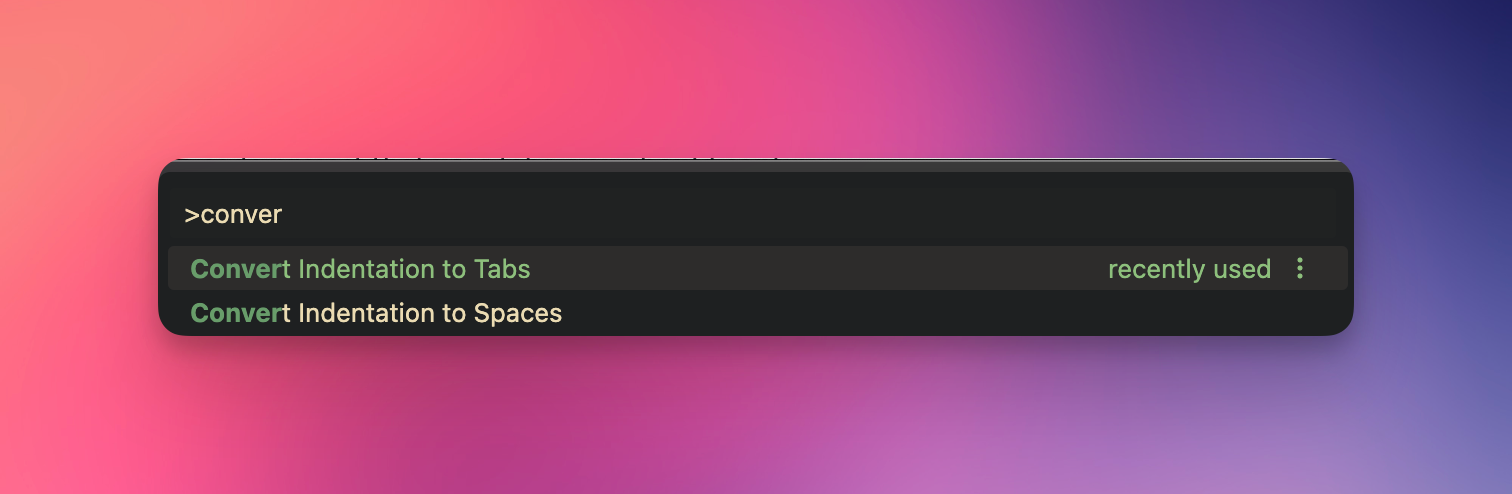
Open your search and replace panel in other text editors such as Sublime or Notepad++.
Search for \t
characters and replace them with four space characters.
Fix 2: Mismatch Indentation Block
Another cause of the indentation error in Python is placing a mismatching indentation.
For example:
x = 100
y = 10
if x > y:
print(x)
else:
print(y)
In this case, the statement does not match its parent if block. Therefore, running the code above returns an indentation error.
You can fix it by aligning your blocks in matching levels.
Closing
In Python, the IndentationError: unindent does not match any outer indentation level
error is caused when you mix tabs and spaces in your code or mismatch indentation levels.